I have been programming SAS for a LONG time and have never seen much in the way of programming standards. For example, most SAS programmers indent DATA and PROC statements (I like three spaces). Most programmers do not like to see more than one statement on a line and most agree that there should be blank lines between program boundaries (DATA and PROC steps).
I thought I would share some of my thoughts on programming standards, with the hope that others will chime in with their ideas.
-
• I like to indent all the statements in a DO group or DO loop. If there are nested groups, each one gets indented as well.
• I prefer variable names in proper case.
• I am not a fan of camel-case. For example, I prefer Weight_Kg to WeightKg. The reason that some programmers like camel-case is that SAS will automatically split a variable name at a capital letter in some headings.
• I like my TITLE statements in open code, not inside a PROC. To me, that makes sense because TITLE statements are global.
• There should be no conversion messages (character to numeric or numeric to character) in the SAS log. For example use Num = INPUT(Char_Num,12.); instead of Num = 1*Char_Num;. The latter statement forces an automatic character to numeric conversion and places a message in the log.
• I always use the statement ODS NOPROCTITLE;. This eliminates the default SAS procedure name at the top of the output.
• Although fewer and fewer people are reading raw text data, I like my @ signs to all line up in my INPUT statement.
• I like to use the /* and */ comments to define all macro variables. For example:
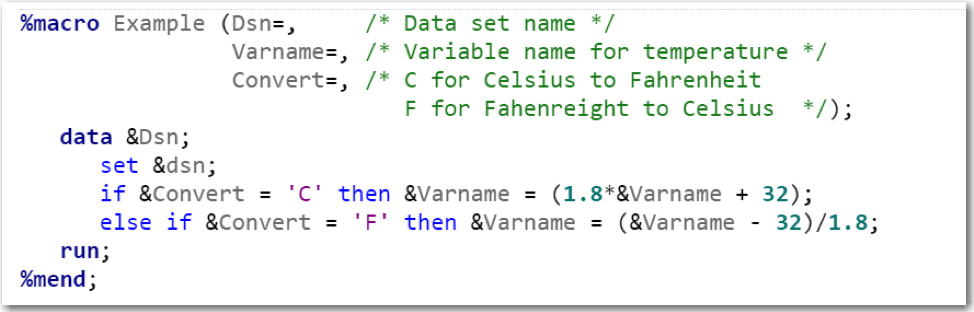
Notice that I prefer named parameters in my macros, instead of positional parameters.
If this seems like too much work - SAS Studio has an automatic formatting tool that can help standardize your programs. For example, look at the code below:
Really ugly, right? Here is how you can use the automatic formatting tool in SAS Studio.
When you click this icon, the program now looks like this:
That’s pretty much the way I would write it. By the way, if you don't like how Studio formatted your code, enter a control-z to undo it.
For more tips on writing code and how to get started in SAS Studio – check out my book, Learning SAS by Example: A Programmer’s Guide, Second Edition. You can also download a free book excerpt. To also learn more about SAS Press, check out the up-and-coming titles, and receive exclusive discounts make sure to subscribe to the SAS Books newsletter.
12 Comments
I like to put a comment right after my procs and data steps to tell me what that does when I collapse all the code blocks. Try to keep it short so it fits on the same line. This way I can collapse all code blocks and the program doesn't feel as overwhelming. EG user and mainly find this helpful where I can collapse the blocks.
+ proc sql; /* this section creates this table*/
+ data want; /* this section does this */
Thanks for the blog and comments everyone. Got a lot out of this.
That is a great idea. I usually don't collapse blocks (my programs are usually not that long), but if I did, I would use your idea. Thanks.
First, appreciate this article, the importance of creating "readable" SAS programs and the comments submitted on this topic so far. As a long time programmer/developer in SAS and many other programming languages as I'm sure many others are as well, there are some fundamentals for writing good programs that should always be adhere too regardless of if you're writing in SAS, Java, Javascript, etc., including:
1. Assume you're not always going to be the person having to run, maintain, etc. the program, so write it as such that someone else can easily understand it.
2. Comments are a good thing, especially if you're doing some type of complex calculation, operation, do-loop, etc. This has saved me more than a few times when I developed some very clever code and then had to modify it a couple of years later.
3. White space, indenting, etc. really helps the general "readability" of code.
4. This more of a Program Editor note, but incredibly help...use the color coding functionality of the SAS Editor to highlight different functions, data types, etc. I have the background color change for Comments and String variables and it's amazing how easy it is to notice you're missing a tick mark, semi-colon or end of comment (*/) mark when your whole program changes color 🙂
5. Make variable names meaningful...yes, it's a few extra keystrokes, but the ability to quickly and easily understand the meaning of a variable is invaluable. (e.g. "X" vs. "LastName")
Hi. Thanks for your comments. Adding comment statements has helped me understand code I wrote years ago. There is a "rule" that after a period of time (6 weeks?) that reading your own code is equivalent to reading someone else's code. It's a shame that so many of the posts showing good programming practices are re-formatted with proportional text (rather than mono-spaced) text. I suggest that you use a screen capture of a piece of code so that it doesn't get re-formatted.
Keep the comments coming.
I would change the code as this for debugging and readability:
Indenting and aligning can be a great way not only to improve readability but also to facilitate debugging. For instance, I like to indent statements within a do loop and line up my END statement with the corresponding DO as shown below. This not only makes the conditional code easily identifiable, it also helps when you have nested DO statements and are trying to keep track of where each ends (also useful for tracking down missing END statements).
From SQL programmers I have learned to vertically align the commas. Like:
* use fixed width font;
And I use it also for some macros aligning the = symbols:
Just my 2 cents.
Eric
I copied this from one of my favorite SAS programmers that always insisted on "neat looking" SAS code. I always put this on top of each of my codes so another programmer can easily learn the "purpose" of the program.
*--------------------------------------------------------------------------------------------*;
* SAS code: [file address]\example.sas *;
* Input(s): [file address]\input_example.xlsx *;
* Output(s): [file address]\output_example.pdf
* Objective: Why are we writing this SAS program? *;
* Date: *;
*---------------------------------------------------------------------------------------------*;
For no real reason, I don't like that style of comment, preferring /* comment */ style.
I've also seen issues when running in batch on Unix if the * comment ; style contains a single apostrophe.
Hi Chris.
You may get two replies from me because I tried earlier and didn't see it.
I tend to use both the * and the /* */ type comments. For adding comments in a program, I tend to use the *. When I write macros, I like to use the /* */ comments because they can be used withing a SAS statement (see my example in the blog). Thanks for your comments. If you have other ideas about SAS programming styles, either leave a comment or email me directly.
Best,
Ron
I try to avoid /* */ comments in the "heart" of my programs because if they're included then you lose the ability to easily comment out a step or block of code. I only use that comment type in my header.
Thanks for this post though, this is great. As much as I keep urging my students, I just can't get them to take program formatting seriously
Bob
P.S. since I'm replying to you, I have to mention that only this week I caught for the first time your little INT/Ent quip in your Learning SAS book. As a lover of Tolkien and an admitted purveyor of rimshot jokes, I have to say nicely done.
I tend to agree, but if I want to comment out a block of code which contains /* … */, I put %macro donotexecute ; … %mend donotexecute; around it.