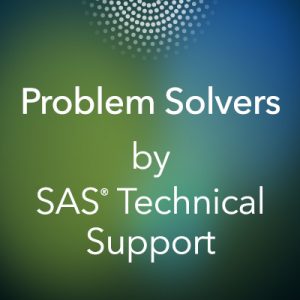
SASPy and the SAS kernel for Jupyter Notebook enable you to connect to SAS® 9.4 and the SAS® Viya® compute server from your client machine. You can then interact with SAS and SAS Viya via Python. If you are not familiar with SASPy and the SAS kernel, this article provides an introduction.
Installing SASPy
SASPy is a client application. You install SASPy on the same client where Python is installed. If SAS is installed on a server, you do not need to install SASPy on the server (because it needs to be installed only on the client).
Since most users install Python, SASPy, and the SAS kernel on a Microsoft Windows client, this article assumes that your client is a Windows client too. But your client could also be a Linux or UNIX environment.
In most situations, installing SASPy on your client machine is as simple as issuing a Python PIP command on your client. For example, if your client is a Windows client, issue this command from a DOS prompt or DOS command line:
pip install saspy |
To verify that SASPy is properly installed, submit the following client command:
pip show saspy |
The information that is displayed by the pip show saspy command also tells you which version of SASPy is installed and the client directory in which SASPy is installed.
Installing the SAS kernel
The SAS kernel is also a client application. You install the SAS kernel on the same client where Python and SASPy are installed. Note that while the use of SASPy does not require the SAS kernel, the SAS kernel does require SASPy to be installed. Also, in order to use the SAS kernel, you must first be able to use SASPy to connect successfully to SAS or SAS Viya.
In most situations, installing the SAS kernel on your client machine is as simple as issuing a Python PIP command. For example, if your client is a Windows client, issue this command from a DOS prompt or DOS command line:
pip install sas_kernel |
To verify that the SAS kernel is properly installed, submit the following client command:
pip show sas_kernel |
The information that is displayed by the pip show sas_kernel command also tells you which version of the SAS kernel is installed and the client directory in which the SAS kernel is installed.
Python and Java dependencies
SASPy and the SAS kernel require Python 3.4 or higher.
Also, most configurations that use SAS 9.4 require that Java be installed on your client. To check to see whether you have Java on your client, issue the following command:
java -version |
Common operating environments
This article discusses several commonly used operating environments that use SASPy and the SAS kernel:
- A local SAS session that is running on Windows, where both SAS and SASPy are installed on the same local Windows client machine
- A remote SAS session that is running on a Windows server
- A remote SAS session that is running on a Linux server
- A SAS Programming Runtime Environment (SPRE) compute server that is running as part of a SAS Viya installation
Connecting to a local SAS session that is running on Windows
A common scenario is to use SASPy with a local installation of SAS on Windows. In this case, use the WINLOCAL definition in your sascfg.py configuration file. The configuration file resides in your SASPy installation directory. To determine the exact name of that directory, issue the following command on your client machine:
pip show saspy |
To use the WINLOCAL definition, find the following line in your sascfg.py file:
SAS_config_names=['default'] |
Modify the line to match the following:
SAS_config_names=['winlocal'] |
To connect to a local Windows installation of SAS, submit the following Python statements:
import saspy import pandas as pd sas = saspy.SASsession(cfgname="winlocal") |
Connecting to a remote SAS session that is running on a Windows server
Another common scenario is to use SASPy on your client machine to connect to SAS 9.4, which is running on a separate Windows server. In this case, use the WINIOMWIN definition in your sascfg.py configuration file.
To use the WINIOMWIN definition, find the following line in your sascfg.py file:
SAS_config_names=['default'] |
Modify the line to match the following:
SAS_config_names=['winiomwin'] |
Then, farther down in your sascfg.py file, find the following default definition:
winiomwin = {'java' : 'java', 'iomhost' : 'windows.iom.host', 'iomport' : 8591, } |
Modify the value for IOMHOST in this definition by specifying the host name of the SAS IOM server that is running on Windows.
Then, to connect to SAS 9.4 on your Windows server, submit the following Python statements from your client:
import saspy import pandas as pd sas = saspy.SASsession(cfgname="winiomwin") |
Connecting to a remote SAS session that is running on a Linux server
You might use SASPy on your client machine to connect to SAS 9.4, which is running on a Linux server. In this case, use the WINIOMLINUX definition in your sascfg.py configuration file.
To use the WINIOMLINUX definition, find the following line in your sascfg.py file:
SAS_config_names=['default'] |
Modify the line to match the following:
SAS_config_names=['winiomlinux'] |
Then, farther down in your sascfg.py file, find the following default definition:
winiomlinux = {'java' : 'java', 'iomhost' : 'linux.iom.host', 'iomport' : 8591, } |
Modify the value for IOMHOST in this definition by specifying the host name of the SAS IOM server that is running on Linux.
Then, to connect to SAS 9.4 on your Linux server, submit the following Python statements from your client:
import saspy import pandas as pd sas = saspy.SASsession(cfgname="winiomlinux") |
Connecting to a SPRE server that is running as part of SAS Viya
SASPy cannot connect directly to a SAS® Cloud Analytic Services (CAS) server that is running as part of SAS Viya. However, SASPy can connect to the SPRE server that is running as part of SAS Viya. (The SPRE server is also referred to as the compute server in SAS Viya.) In this case, use the HTTPVIYA definition in your sascfg.py configuration file.
To use the HTTPVIYA definition, find the following line in your sascfg.py file:
SAS_config_names=['default'] |
Modify the line to match the following:
SAS_config_names=['httpviya'] |
Then, farther down in your sascfg.py file, find the following default definition:
httpviya = {'ip' : 'name.of.your.spre.server', 'ssl' : False, # this will use port 80 'context' : 'Data Mining compute context', 'authkey' : 'viya_user-pw', 'options' : ["fullstimer", "memsize=1G"] } |
Modify the IP value in this definition by specifying the name of the SPRE server for your installation of SAS Viya.
Then, to connect to the SPRE server, submit the following Python statements from your client:
import saspy import pandas as pd sas = saspy.SASsession(cfgname="httpviya") |
Using SASPy
After you connect successfully to SAS or the SPRE server, you can then submit Python statements via your client to interact with SAS. For example, you can display the first five rows (observations) in SASHELP.CLASS by submitting the following statements:
cars = sas.sasdata("cars","sashelp") cars.head() |
You can display summary statistics for SASHELP.CLASS with the following code:
cars = sas.sasdata("cars","sashelp") cars.describe() |
Using the SAS kernel for Jupyter Notebook
The SAS kernel enables you to use the Jupyter Notebook interface to execute SAS code and view results inline.
As previously noted, the SAS kernel requires SASPy to be installed. You also must be able to connect successfully to SAS or SAS Viya via SASPy. After you do so, after bringing up Jupyter Notebook, in the upper right-hand corner of your notebook, choose New > SAS.
After that, you can then submit regular SAS statements via Jupyter Notebook. Here is an example:
proc print data=sashelp.class; run; |
Additional resources and documentation
For additional information about using SASPy and the SAS kernel, see these resources: