I created this project as a fun exercise to emulate the popular game Wordle in the SAS language. I was inspired by this story about a GitHub user who implemented Wordle in a Bash shell. (See the Bash script here. Read the comments -- it's an amazing stream of improved versions, takes in different programming languages, and "code golf" to reduce the lines-of-code.)
While developing my SAS solution, I created a Twitter poll to ask how other SAS programmers might approach it.
For me it was always going to be arrays, since that's what I know best. I'd love to be able to dash out a hash object approach or even use SAS/IML, but it would take me too long to wrap my brain around these. The PRX* regex function choice is bit of a feint -- regular expressions seem like a natural fit (pattern matching!), but Wordle play has nuances that makes regex less elegant than you might guess. Prove me wrong!
Two SAS users from Japan, apparently inspired by my poll, each implemented their own games! I've added links to their work at the end of this article.
How to code Wordle in SAS
You can see my complete SAS code for the game here: sascommunities/wordle-sas. I've also described my coding approach in more detail on the SAS Community Library.
The interesting aspects of my version of the game include:
- Uses the "official" word lists from New York Times as curated by cfreshman. I found these while examining the Bash script version. I used PROC HTTP to pull this list dynamically.
- Also verifies guesses as "valid" using the list of allowed guesses, again curated by cfreshman. You know you can't submit just any 5 characters as a guess, right? That's an important part of the game.
- Uses DATA step array to verify guesses against solution word.
- Use DATA step object method to create a gridded output of the game board. Credit to my SAS friends in Japan for this idea!
I've added a screenshot of example game play below. This was captured in SAS Studio running in SAS Viya (and yes, I realize this example doesn't represent a well-played game).
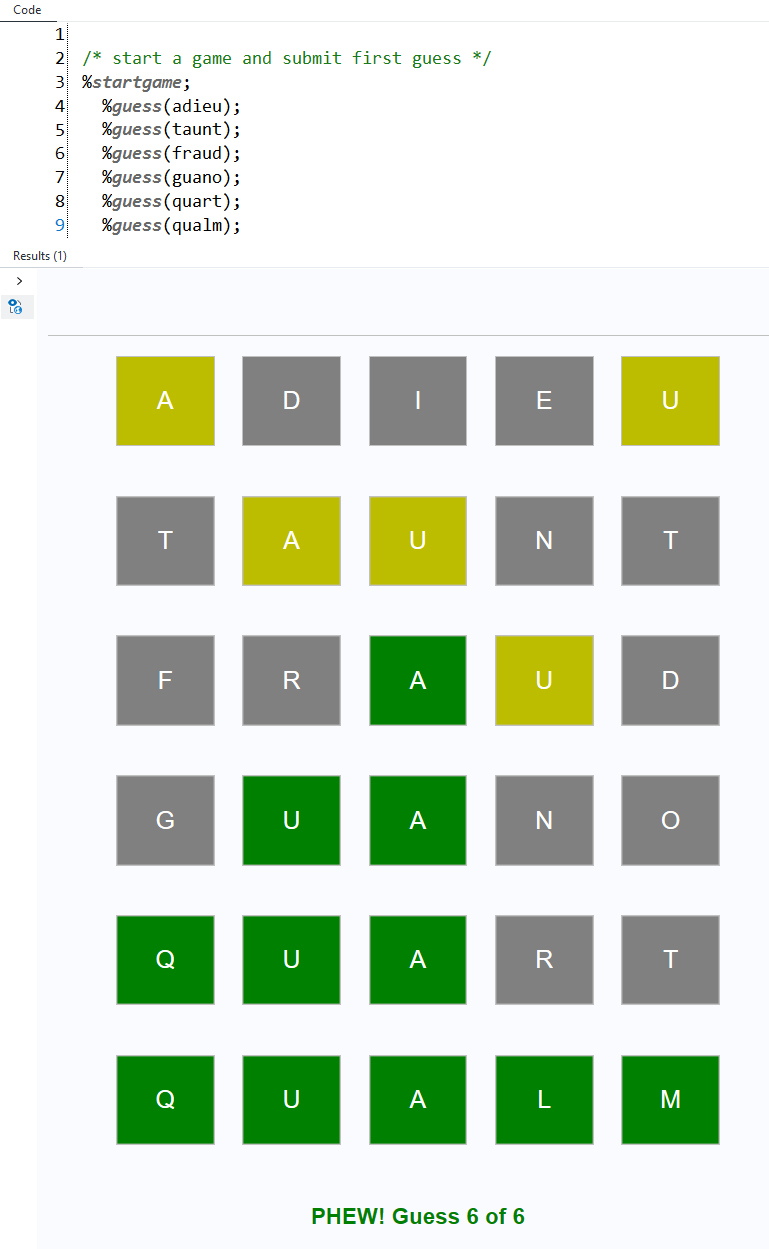
How to play Wordle in SAS
To play:
- Submit the wordle-sas.sas program in your SAS session. This program should work in PC SAS, SAS OnDemand for Academics, SAS Enterprise Guide, and SAS Viya.
The program will fetch word lists from GitHub and populate into data sets. It will also define two macros you will use to play the game.
- Start a game by running:
%startGame;
This will select a random word from the word list as the "puzzle" word and store it in a SAS macro variable (don't peek!) - Optionally seed a game with a known word by using an optional 5-character word parameter:
%startGame(crane);
This will seed the puzzle word ("crane" in this example). It's useful for testing. See a battery of test "sessions" in wordle-sas-tests.sas - Submit a first guess by running:
%guess(adieu);
This will check the guess against the puzzle word, and it will output a report with the familiar "status" - letters that appear in the word (yellow) and that are in the correct position (green). It will also report if the guess is not a valid guess word, and it won't count that against you as one of your 6 permitted guesses.
Use the %guess() macro (one at a time) to submit additional guesses. The program keeps track of your guesses and when you solve it, it shares the familiar congratulatory message that marks the end of a Wordle session. Ready to play again? Use the %startGame macro to reset.
Start a fresh game using Git functions
If you don't want to look at or copy/paste the game code, you can use Git functions in SAS to bring the program into your SAS session and play. (These Git functions require at least SAS 9.4 Maint 6 or SAS Viya.)
options dlcreatedir; %let repopath=%sysfunc(getoption(WORK))/wordle-sas; libname repo "&repopath."; data _null_; rc = gitfn_clone( "https://github.com/sascommunities/wordle-sas", "&repoPath." ); put 'Git repo cloned ' rc=; run; %include "&repopath./wordle-sas.sas"; /* start a game and submit first guess */ %startGame; %guess(adieu); |
Suggestions?
I know that my program could be more efficient...perhaps at the cost of readability. Also it's possible that I have some lingering bugs, although I did quite a bit of testing and bug-fixing along the way. Pro tip: The DATA step debugger (available in SAS Enterprise Guide and in SAS Viya version of SAS Studio) was a very useful tool for me!
Your feedback/improvements are welcome. Feel free to comment here or on the GitHub project.
Wordle games in SAS by other people
SAS users in Japan quickly implemented their own versions of Wordle-like games. Check them out:
- SASでWORDLE by user japelin. It uses PROC REPORT to output the game grid, similar to my first version. See SASでWORDLE(その2:UI追加)for a version with a simple UI.
- WordleをSASで実装してみた.判定はdo overとhash iterator object), 出力はSAS RWIを使ってみた by user sasyupi. This version uses the hash object in DATA step -- efficient! It also uses the DATA step object to output the game grid for display, and I borrowed that technique in my revised version.
- SASでWORDLE(その3:SAS/AF) also by user japelin. It's a SAS/AF application that you can run if you have SAS for Windows on your desktop.
11 Comments
Excellent work Chris! I recently got onboard with Wordle so this was fun to read and try out...and see the power of Base SAS once again!
Hi Chris! I'm an MPH grad student who is very new to SAS and this was the first time I've used SAS for something for fun that's not analyzing datasets! Thanks for the little respite from my schoolwork.
Analyzing data sets is fun! Right?
Nice job Chris. This reminds me of a time “long, long, ago”, well really the late 1990s early 2000s when I wrote a SAS/AF and SCL battleship game as part of a testing routine. BTW: This application still exists in my unix directory and still works whenever I check it every few years.
Many years ago Mark Paschalidis made a "Castle Wolfenstein" clone called "Wolfensas". It still works on 32-bit SAS for Windows (a rare installation these days).
This is the first time I will it
First time for Wordle? Welcome to the world of the addicted.
Very fun!
Is Wordle becoming the next level of challenge beyond "hello world"?
It's definitely a good comp sci exercise and a way to learn your way around a programming language. "Build Wordle in it!"
Chris did a great job! I just started using Wordle, so it was fun to read and experiment with this and once more realize the power of Base SAS